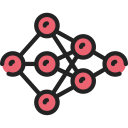
Data Structures
Data Structures & Algorithms
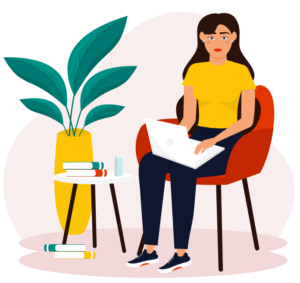
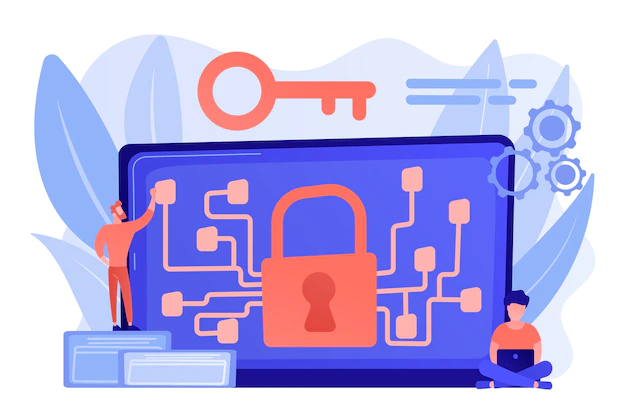
Data Structures are the programmatic way of storing data so that data can be used efficiently. Almost every enterprise application uses various types of data structures in one or the other way. This tutorial will give you a great understanding on the Data Structures needed to understand the complexity of enterprise-level applications and the need of algorithms, and data structures.
We will also be solving lots and lots of questions from websites like LeetCode, CodingBat, and HackerRank to improve our understanding and problem-solving skills.
Basic Python Crash Course
➔ Intro to Algorithms using Simple Programs/Concepts :
◆ Narcissistic Number
◆ Reversing a Number
◆ Making a Tic Tac Toe Game using Dictionaries
◆ Fibonacci Series
◆ Recursion
Algorithm Analysis and Big O
◆ Time complexity
◆ Space complexity
◆ Analysis of iterative algorithm
◆ Analysis of recursive algorithm
◆ Analysis of common loops
◆ Examples
Data Structures and their Implementation
➢ Left rotation of array by 1
➢ Left rotation of array by d places
➢ Maximum consecutive 1s
➢ Maximum difference problem
➢ Sliding window technique
➢ More problems
Dictionaries
➢ Dictionary with maximum count of pairs
➢ Convert dictionary to k-sized dictionary
➢ All substrings frequency in a string
➢ Practice problems
Linked Lists
➢ Comparison with array
➢ Detecting loops
➢ Deleting nth node in linked list
➢ Iterative and recursive methods to reverse a linked list.
➢ Merge two sorted linked lists
➢ Segregating even odd nodes of linked list
➢ Practice problems
Stacks
➢ Applications
➢ Balanced parenthesis
➢ Previous greater element
➢ Next greater element
➢ Practice problems
Queue
➢ Stack using queue
➢ Reversing a queue
➢ Practice problems
➢ Dequeue
Trees
➢ Binary Tree
➢ Tree traversals(inorder, postorder, preorder, level order)
➢ Size of binary tree
➢ Convert binary tree into doubly linked list
➢ Check for balanced binary tree
➢ Binary Search Tree
➢ Insertion, deletion in BST
➢ Self balancing BST
➢ Practice problems
Graphs
➢ Adjacency matrix
➢ Breadth-First Search
➢ Depth-first search
➢ Shortest path
➢ Prims Algorithm
➢ Dijkstra’s shortest path algorithm
➢ Bellman-Ford shortest path algorithm
➢ Bridges in graph
➢ Practice problems
Heaps
➢ Heap sort
➢ Priority Queue
➢ Sort K-sorted array
➢ K-largest elements
➢ Merge k-sorted arrays
➢ Practice problems
Searching and Sorting Algorithms and their Implementation
◆ Binary Search
◆ Insertion Sort
◆ Selection Sort
◆ Bubble Sort
◆ Merge Sort
◆ Quick Sort
◆ Practice problems on all the sorting techniques
Algorithms Technique
➢ Introduction
➢ Activity selection problem
➢ Fractional knapsack
➢ Job sequencing problem
➢ Practice problems
◆ Dynamic Programming
➢ Memoization
➢ Tabulation
➢ Longest common subsequence
➢ Coin change count combinations
➢ Maximum coins to make a value
➢ Optimal strategy for a game
➢ Maximum jumps to reach the end
➢ 0-1 knapsack problem
➢ More problems
◆ Backtracking
➢ Introduction
➢ Rat in a maze
➢ N Queen problem
➢ Sudoku problem
➢ More problems
Who are MasterJi’s Instructors?
Our instructors are subject matter experts from top universities. Instructors are highly-vetted and background checked prior to joining and undergo extensive training before ever teaching on our platform.
With highly qualified, interactive, and experienced faculty, learning becomes engaging and effective. We at MasterJi aspire to take teaching to a new benchmark where skills are imparted in a unique way by building a super friendly teacher-student relation and at optimum prices.
For more information mail us at: [email protected]
This course includes
We in MasterJi classes believe that making real life projects is the best way to test your learning. For every course we encourage our students to build projects and enhance their learning experience
1-1 live sessions : Live sessions offer opportunities for interaction between students and with the instructor which in turn proves to be beneficial for better understanding and learning experience
After the completion of every level we provide Certificate of completion.
Completion of Python Level 1
Sign Up For A Free Trial
Our Fee Structure
For individuals choosing multiple classes per week, we provide the opportunity to enroll in additional classes at a discounted rate across all levels. The tuition for each additional once-a-week class is just $140 for a 4-week period.